How Builders Can Make improvements to Their Debugging Techniques By Gustavo Woltmann
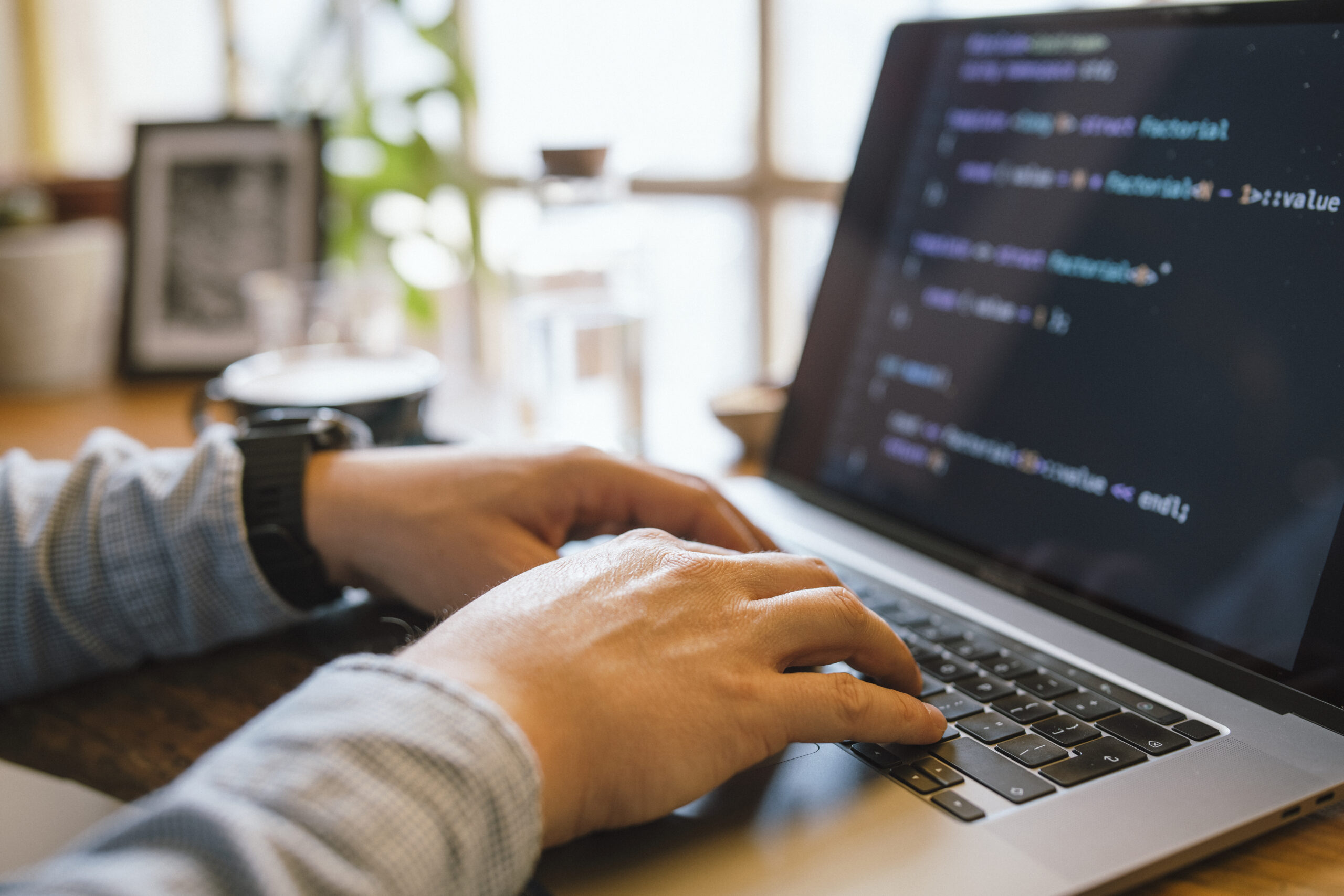
Debugging is one of the most essential — nevertheless generally overlooked — abilities within a developer’s toolkit. It's actually not just about fixing broken code; it’s about comprehending how and why items go Mistaken, and Mastering to think methodically to solve issues efficiently. Regardless of whether you're a newbie or possibly a seasoned developer, sharpening your debugging competencies can help you save several hours of annoyance and considerably transform your productivity. Here are several procedures that will help builders degree up their debugging video game by me, Gustavo Woltmann.
Grasp Your Equipment
One of many quickest means builders can elevate their debugging capabilities is by mastering the instruments they use every single day. Although writing code is one Element of growth, figuring out tips on how to communicate with it successfully all through execution is Similarly essential. Modern day development environments occur Outfitted with powerful debugging abilities — but several developers only scratch the area of what these equipment can perform.
Just take, as an example, an Built-in Advancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments permit you to established breakpoints, inspect the value of variables at runtime, action by code line by line, and also modify code on the fly. When utilised properly, they Permit you to observe accurately how your code behaves all through execution, and that is priceless for tracking down elusive bugs.
Browser developer instruments, including Chrome DevTools, are indispensable for entrance-close developers. They assist you to inspect the DOM, keep an eye on community requests, check out serious-time performance metrics, and debug JavaScript from the browser. Mastering the console, resources, and network tabs can switch frustrating UI concerns into workable duties.
For backend or method-stage builders, tools like GDB (GNU Debugger), Valgrind, or LLDB offer you deep Command about operating processes and memory administration. Discovering these tools might have a steeper Mastering curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Beyond your IDE or debugger, turn out to be relaxed with Model Command systems like Git to be familiar with code history, discover the exact second bugs have been launched, and isolate problematic changes.
Eventually, mastering your instruments suggests likely further than default configurations and shortcuts — it’s about developing an intimate understanding of your growth natural environment to make sure that when challenges crop up, you’re not shed at midnight. The better you realize your resources, the more time you are able to invest solving the actual issue instead of fumbling via the process.
Reproduce the Problem
One of the most critical — and sometimes ignored — actions in effective debugging is reproducing the problem. Right before leaping to the code or building guesses, builders want to create a consistent ecosystem or circumstance in which the bug reliably appears. With out reproducibility, correcting a bug will become a match of opportunity, often bringing about squandered time and fragile code adjustments.
The first step in reproducing a challenge is collecting just as much context as is possible. Request inquiries like: What actions triggered The problem? Which environment was it in — progress, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The greater detail you have got, the less complicated it gets to be to isolate the precise circumstances less than which the bug takes place.
After you’ve gathered adequate information and facts, try and recreate the issue in your neighborhood surroundings. This may suggest inputting the same knowledge, simulating similar consumer interactions, or mimicking procedure states. If The problem appears intermittently, take into account crafting automated assessments that replicate the edge circumstances or point out transitions concerned. These checks not only support expose the problem but in addition protect against regressions in the future.
In some cases, the issue could possibly be ecosystem-particular — it would transpire only on certain working systems, browsers, or below distinct configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms could be instrumental in replicating these kinds of bugs.
Reproducing the condition isn’t just a stage — it’s a attitude. It involves tolerance, observation, and a methodical technique. But when you finally can continuously recreate the bug, you're currently halfway to fixing it. Having a reproducible circumstance, You may use your debugging applications more successfully, check possible fixes securely, and talk much more Obviously using your crew or end users. It turns an abstract grievance into a concrete problem — Which’s the place developers thrive.
Read and Understand the Mistake Messages
Mistake messages in many cases are the most valuable clues a developer has when something goes wrong. Rather then looking at them as discouraging interruptions, developers ought to understand to deal with error messages as immediate communications through the program. They frequently tell you what precisely happened, wherever it took place, and occasionally even why it happened — if you know the way to interpret them.
Commence by studying the message carefully As well as in total. Numerous builders, particularly when under time force, glance at the very first line and straight away start out producing assumptions. But further while in the error stack or logs may well lie the correct root induce. Don’t just duplicate and paste error messages into search engines — examine and comprehend them to start with.
Split the mistake down into components. Is it a syntax error, a runtime exception, or a logic error? Will it level to a selected file and line variety? What module or function brought on it? These queries can guideline your investigation and level you towards the responsible code.
It’s also handy to know the terminology with the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java frequently observe predictable patterns, and Finding out to acknowledge these can significantly hasten your debugging process.
Some glitches are imprecise or generic, and in People conditions, it’s essential to look at the context wherein the error transpired. Look at associated log entries, input values, and up to date variations within the codebase.
Don’t forget about compiler or linter warnings possibly. These frequently precede more substantial challenges and supply hints about possible bugs.
Eventually, mistake messages are certainly not your enemies—they’re your guides. Learning to interpret them properly turns chaos into clarity, serving to you pinpoint challenges quicker, minimize debugging time, and become a far more successful and self-assured developer.
Use Logging Sensibly
Logging is One of the more strong instruments in a very developer’s debugging toolkit. When applied correctly, it offers serious-time insights into how an software behaves, encouraging you realize what’s taking place under the hood without needing to pause execution or stage through the code line by line.
A great logging technique begins with understanding what to log and at what stage. Widespread logging stages incorporate DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for specific diagnostic facts all through enhancement, Details for standard functions (like productive begin-ups), WARN for opportunity difficulties that don’t crack the appliance, ERROR for precise challenges, and Deadly when the system can’t go on.
Prevent flooding your logs with abnormal or irrelevant knowledge. An excessive amount of logging can obscure important messages and decelerate your program. Focus on vital gatherings, condition adjustments, enter/output values, and significant choice details within your code.
Format your log messages Plainly and regularly. Involve context, for example timestamps, request IDs, and performance names, so it’s simpler to trace issues in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs let you track how variables evolve, what problems are met, and what branches of logic are executed—all with no halting the program. They’re Specially valuable in creation environments where stepping by way of code isn’t possible.
Also, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about balance and clarity. That has a nicely-imagined-out logging method, you may lessen the time it will take to identify challenges, acquire deeper visibility into your apps, and Increase the General maintainability and trustworthiness of your respective code.
Think Like a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To properly detect and resolve bugs, builders ought to solution the process like a detective solving a thriller. This frame of mind can help stop working sophisticated troubles into workable sections and abide by clues logically to uncover the foundation cause.
Commence by collecting evidence. Consider the indications of the problem: error messages, incorrect output, or overall performance problems. Much like a detective surveys a crime scene, gather as much related info as you may devoid of leaping to conclusions. Use logs, take a look at situations, and consumer studies to piece with each other a clear picture of what’s going on.
Subsequent, type hypotheses. Ask yourself: What could be producing this actions? Have any improvements not long ago been manufactured for the codebase? Has this challenge transpired prior to under identical situation? The purpose is always to narrow down possibilities and detect probable culprits.
Then, exam your theories systematically. Try and recreate the trouble in a managed setting. In the event you suspect a selected purpose or element, isolate it and validate if The difficulty persists. Just like a detective conducting interviews, ask your code issues and Allow the results direct you closer to the reality.
Spend shut focus to tiny details. Bugs generally hide from the least envisioned areas—similar to a missing semicolon, an off-by-a person error, or perhaps a race affliction. Be thorough and client, resisting the urge to patch the issue devoid of totally knowledge it. Short term fixes may conceal the actual challenge, only for it to resurface later on.
Last of all, preserve notes on Anything you attempted and uncovered. Equally as detectives log their investigations, documenting your debugging method can help save time for future troubles and assist Some others understand your reasoning.
By pondering just like a detective, builders can sharpen their analytical abilities, strategy challenges methodically, and become simpler at uncovering hidden concerns in advanced systems.
Compose Assessments
Producing checks is among the most effective approaches to increase your debugging competencies and overall growth effectiveness. Checks don't just help catch bugs early but additionally serve as a safety Internet that provides you self esteem when making improvements towards your codebase. A well-tested application is simpler to debug as it helps you to pinpoint precisely in which and when a difficulty happens.
Get started with device assessments, which center on unique capabilities or modules. These smaller, isolated assessments can speedily reveal no matter whether a certain piece of logic is Performing as predicted. Each time a check fails, you right away know exactly where to appear, significantly reducing some time expended debugging. Device exams are Specifically valuable for catching regression bugs—troubles that reappear right after previously being preset.
Upcoming, integrate integration tests and end-to-conclusion exams into your workflow. These assist make certain that various aspects of your application work alongside one another efficiently. They’re especially practical for catching bugs that arise in sophisticated systems with many elements or providers interacting. If a little something breaks, your assessments can tell you which Component of the pipeline failed and less than what situations.
Writing assessments also forces you to Consider critically about your code. To test a characteristic thoroughly, you may need to understand its inputs, predicted outputs, and edge cases. This amount of understanding In a natural way leads to higher code composition and fewer bugs.
When debugging a concern, creating a failing take a look at that reproduces the bug may be a strong starting point. Once the examination fails consistently, you'll be able to focus on fixing the bug and look more info at your exam pass when The problem is resolved. This strategy makes certain that the same bug doesn’t return Later on.
Briefly, crafting tests turns debugging from a annoying guessing activity into a structured and predictable method—supporting you capture a lot more bugs, more rapidly plus more reliably.
Take Breaks
When debugging a tricky concern, it’s effortless to be immersed in the condition—staring at your screen for hours, making an attempt Resolution immediately after Answer. But Just about the most underrated debugging equipment is actually stepping away. Using breaks will help you reset your head, lower annoyance, and infrequently see The difficulty from the new point of view.
When you are way too near the code for far too extensive, cognitive exhaustion sets in. You would possibly get started overlooking noticeable glitches or misreading code that you choose to wrote just several hours before. With this condition, your brain gets to be much less productive at difficulty-solving. A brief wander, a espresso break, or perhaps switching to a different endeavor for ten–15 minutes can refresh your concentrate. Numerous builders report acquiring the foundation of a challenge once they've taken time to disconnect, permitting their subconscious get the job done while in the track record.
Breaks also assist reduce burnout, In particular for the duration of for a longer time debugging sessions. Sitting down in front of a screen, mentally trapped, is not merely unproductive but additionally draining. Stepping absent lets you return with renewed Power and a clearer way of thinking. You could suddenly detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
In the event you’re trapped, a superb rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then take a five–10 moment break. Use that point to move all over, stretch, or do anything unrelated to code. It may sense counterintuitive, Specially under restricted deadlines, but it really truly causes more quickly and more practical debugging In the end.
Briefly, taking breaks is just not an indication of weakness—it’s a wise system. It gives your brain space to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight That always blocks your progress. Debugging can be a psychological puzzle, and relaxation is part of fixing it.
Study From Each Bug
Each and every bug you face is a lot more than simply A short lived setback—it's an opportunity to expand for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural challenge, every one can instruct you some thing useful in case you go to the trouble to reflect and analyze what went Incorrect.
Start out by inquiring yourself a couple of important queries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with better practices like unit tests, code reviews, or logging? The answers often expose blind spots inside your workflow or knowing and enable you to Construct more powerful coding behavior shifting forward.
Documenting bugs can also be an excellent habit. Continue to keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, how you solved them, and Everything you discovered. As time passes, you’ll start to see patterns—recurring issues or popular faults—you can proactively keep away from.
In group environments, sharing Everything you've learned from the bug using your peers is usually In particular strong. Regardless of whether it’s through a Slack information, a short compose-up, or a quick know-how-sharing session, supporting Other people steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In fact, several of the best builders are not those who write best code, but those who repeatedly discover from their faults.
In the end, Just about every bug you repair adds a new layer in your talent set. So up coming time you squash a bug, have a moment to mirror—you’ll occur away a smarter, additional able developer due to it.
Summary
Improving upon your debugging expertise can take time, practice, and persistence — although the payoff is large. It tends to make you a more successful, confident, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a possibility to be better at Everything you do.